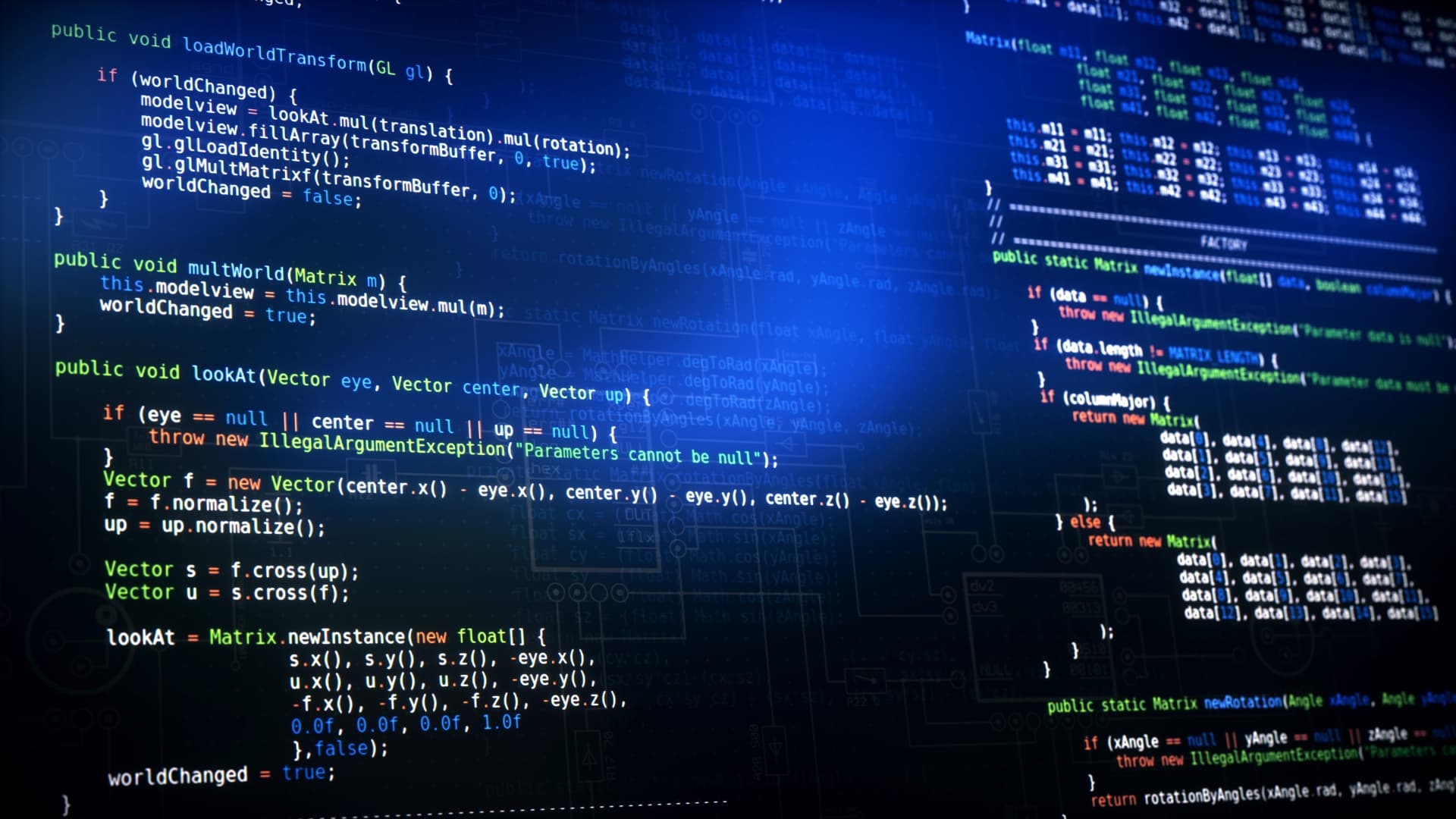
Java Course Syllabus
Introduction to Java and OOPS
- Java Tokens- Comments, Identifiers, Keywords, Separators
- Working with Java Editor Softwares – Editplus, NetBeans, Eclipse
- Packages with static imports
- Working with jar
Modifiers, Datatypes, and Control Flow
- Modifiers – File level, Access level and Non-access level
- Datatypes, Literals, Variables, Type Conversion, Casting & Promotion
- Reading runtime values from keyboard and Properties File
- Operators and Control Statements
Methods, Variables, and Constructors
- Method and Types of methods
- Variable and Types of Variables
- Constructor and Types of constructors
- Block and Types of Blocks
Execution Flow and Architecture
- Declarations, Invocations and Executions
- Compiler & JVM Architecture with Reflection API
- Static Members and their execution control flow
- Non-Static Members and their execution control flow
- Final Variables and their rules
OOP Principles and Advanced Concepts
- Classes and Types of classes
- OOPS- Fundamentals, Models, Relations and Principles
- Coupling and Cohesion (MVC and LCRP Architectures)
- Types of objects & Garbage Collection
- Arrays and Var-arg types
- Enum and Annotation
Design Patterns and Java API
- Design Patterns
- Java API and Project
- API and API Documentation
- Fundamental Classes – Object, Class, System, Runtime
- String Handling
Advanced Java Features
- Exception Handling and Assertions
- Multithreading with JVM Architecture
- IO Streams (File IO)
- Wrapper Classes with Auto boxing and unboxing
- Collections with Generics
- Java 5, 6, 7, 8 new features
- Inner classes
Hibernate and ORM
- Hibernate Training Course Overview
- Advantages of Hibernate compared to JDBC
- Introduction to ORM (Object Relational Mapping)
- Hibernate Resources
- Configuration file
- Mapping file
- Persistent class or POJO
- Client application
- Hibernate Architecture
- Installation and Directory Structure
Java Syllabus Courses
1. Introduction to Java and OOPS
2. Modifiers, Datatypes, and Control Flow
3. Methods, Variables, and Constructors
4. Execution Flow and Architecture
5. OOP Principles and Advanced Concepts
6. Design Patterns and Java API
7. Advanced Java Features
8. Hibernate and ORM