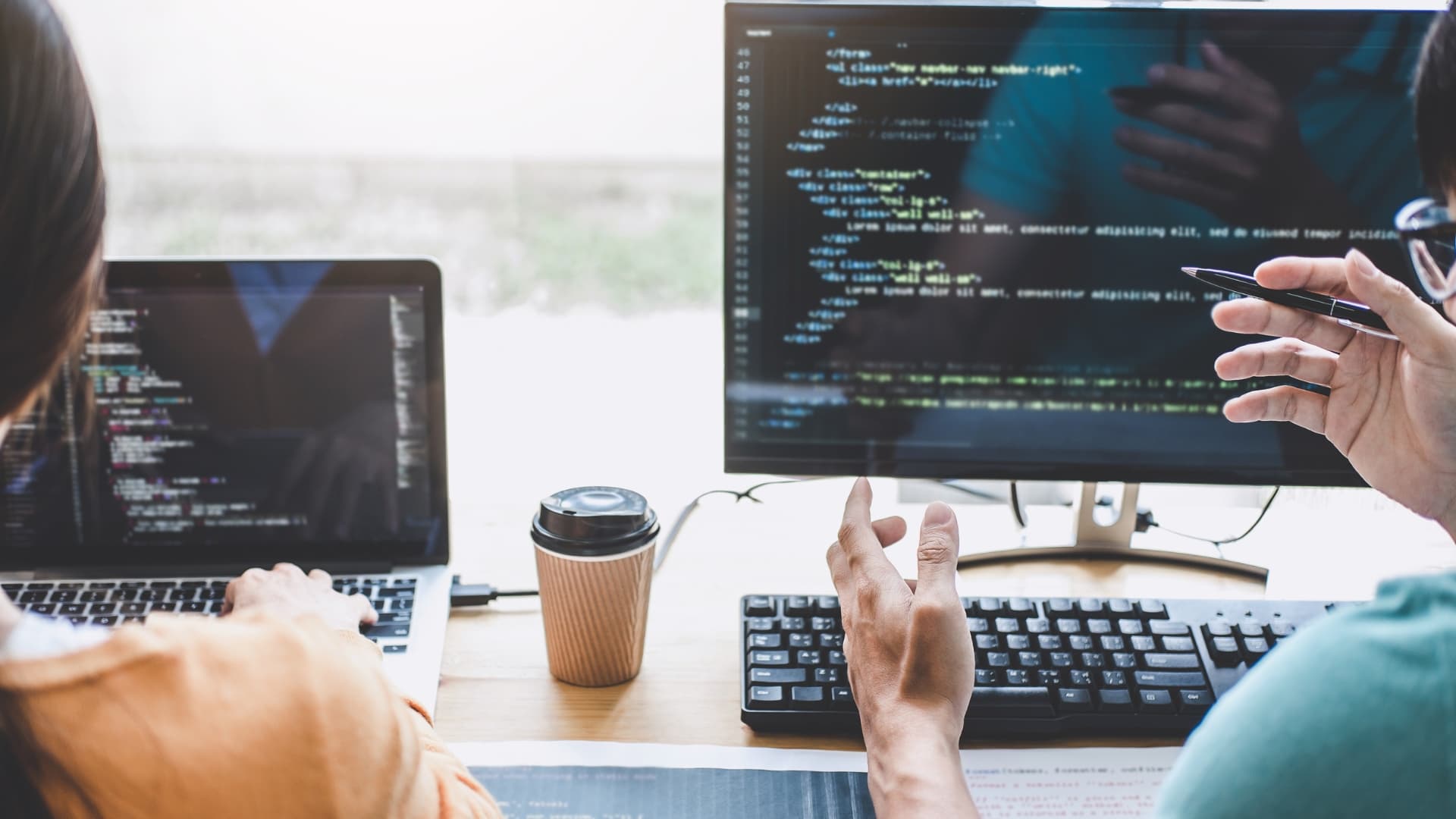
.NET Course Syllabus
Introduction to .NET
- Overview of .NET Framework and .NET Core
- Differences between .NET Framework, .NET Core, and .NET 5/6
- Introduction to Common Language Runtime (CLR) and Common Type System (CTS)
- .NET Standard and its significance
- Understanding the .NET ecosystem (NuGet, libraries, tools)
Programming with C#
- Introduction to C# language
- Data types, variables, and operators
- Control structures (if, switch, loops)
- Methods and parameters
- OOP concepts: Classes and objects, Inheritance, Polymorphism, Encapsulation
- Exception handling
- Collections (arrays, lists, dictionaries)
Advanced C# Concepts
- Delegates and events
- Lambda expressions
- LINQ (Language Integrated Query)
- Asynchronous programming with async/await
- Generics in C#
- Attributes and reflection
Building Desktop Applications
- Introduction to Windows Forms
- Creating a Windows Forms application
- Working with controls and events
- Data binding in Windows Forms
- Introduction to WPF (Windows Presentation Foundation)
- XAML basics and layout controls
- MVVM (Model-View-ViewModel) design pattern
Web Development with ASP.NET
- Introduction to ASP.NET Core
- Creating your first ASP.NET Core application
- MVC (Model-View-Controller) architecture
- Routing and middleware
- Working with views and layouts
- Form handling and validation
- Dependency injection in ASP.NET Core
Web API Development
- Introduction to RESTful services
- Creating and configuring Web APIs with ASP.NET Core
- Routing, controllers, and action methods
- Working with JSON and XML
- Authentication and authorization (JWT, OAuth)
- Versioning APIs
- Testing APIs with Postman or Swagger
Data Access in .NET
- Introduction to Entity Framework Core
- Code-first vs. database-first approaches
- Database migrations and seeding
- CRUD operations with Entity Framework
- Working with SQL Server
- Introduction to Dapper as an alternative ORM
Front-End Development (Optional)
- Overview of front-end technologies (HTML, CSS, JavaScript)
- Introduction to Blazor
- Building interactive web applications with Blazor
- State management in Blazor
Cloud and Deployment
- Introduction to Azure services
- Deploying .NET applications to Azure
- Understanding Azure App Services
- Introduction to Azure Functions
- Monitoring and logging applications
Best Practices and Design Patterns
- Introduction to software design principles (SOLID, DRY, KISS)
- Common design patterns in .NET (Repository, Unit of Work, Singleton)
- Testing methodologies (unit testing, integration testing)
- Introduction to xUnit or NUnit for testing
- Continuous integration and continuous deployment (CI/CD)
.NET Syllabus
1. Introduction to .NET
2. Programming with C#
3. Advanced C# Concepts
4. Building Desktop Applications
5. Web Development with ASP.NET
6. Web API Development
7. Data Access in .NET
8. Front-End Development (Optional)
9. Cloud and Deployment
10. Best Practices and Design Patterns