Questions Courses
1. What is Python?
2. What are Python’s key features?
3. How is memory managed in Python?
4. What are Python’s built-in data types?
5. What is a list, and how is it different from a tuple?
6. What is PEP 8?
7. What is the difference between append() and extend() in Python?
8. What are *args and **kwargs?
9. What is a lambda function?
10. What is the difference between shallow copy and deep copy?
11. How is exception handling done in Python?
12. What is the difference between is and ==?
13. What are Python decorators?
14. What is a generator in Python?
15. What is a module in Python?
16. How do you create a virtual environment in Python?
17. What is GIL (Global Interpreter Lock)?
18. What is a Python class and how do you create one?
19. What is the difference between __str__() and __repr__()?
20. What is list comprehension in Python?
21. What is the difference between a function and a method?
22. What is the difference between remove(), pop(), and del in lists?
23. How can you handle files in Python?
24. What is monkey patching in Python?
25. What are Python metaclasses?
26. What is the pass statement?
27. How can you manage packages in Python?
28. What is the difference between Python 2 and Python 3?
29. What is the use of super() in Python?
30. How do you handle multiple exceptions in Python?
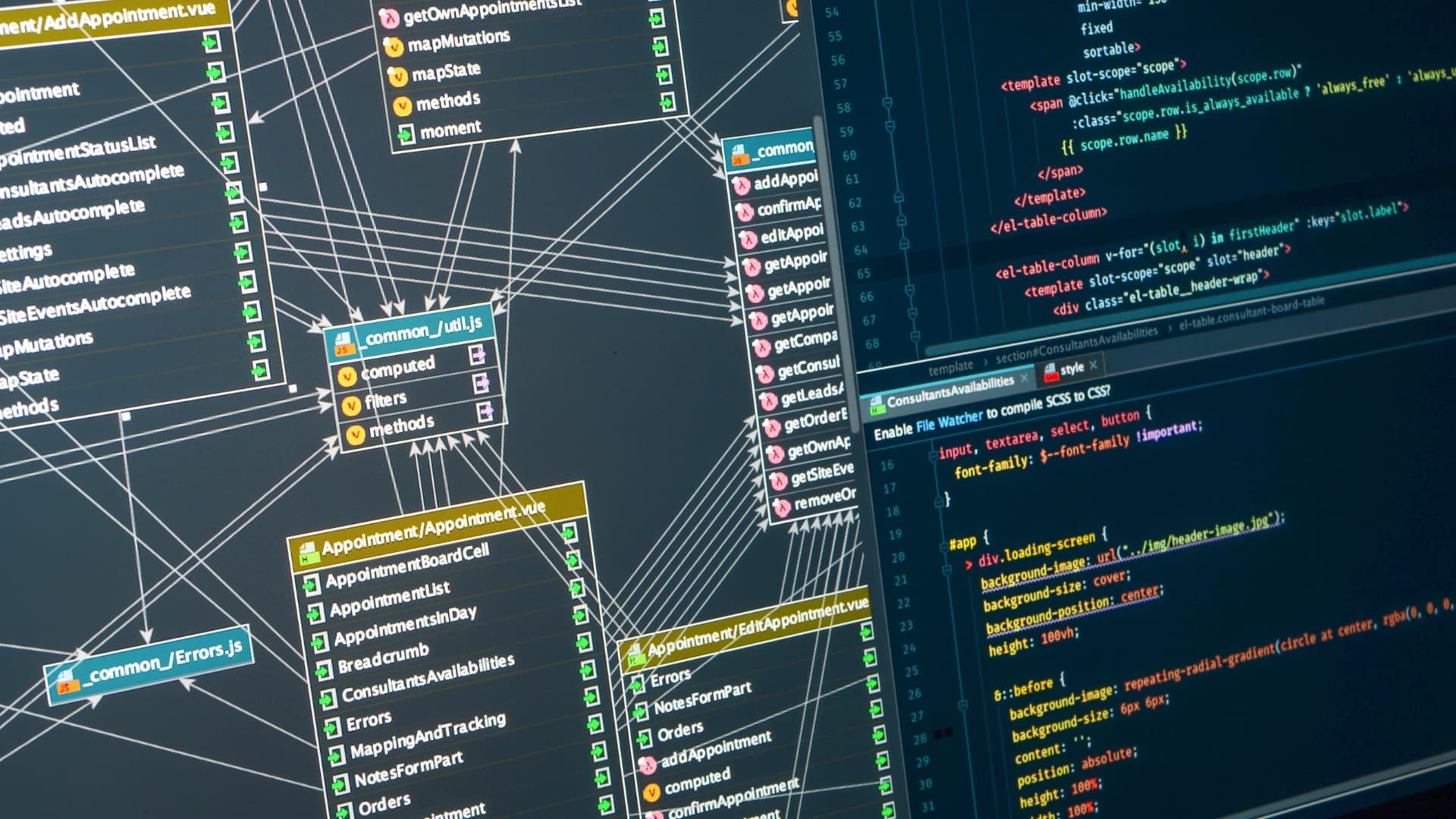
Python Interview Questions
Are you preparing for a Python interview? Here are 30 essential questions to help you succeed in your interview and demonstrate your mastery of Python.
Top Python Interview Questions & Answers
1. What is Python?
- Python is a high-level, interpreted programming language known for its simplicity and readability.
- It supports multiple programming paradigms including object-oriented, functional, and procedural programming.
2. What are Python’s key features?
- Simple and easy to learn
- Interpreted language
- Object-oriented
- Dynamically typed
- Extensive libraries and frameworks
- Cross-platform
3. How is memory managed in Python?
- Memory management in Python is handled by the Python memory manager.
- It includes private heap, garbage collection, and reference counting.
4. What are Python’s built-in data types?
- Numeric: int, float, complex
- Sequences: list, tuple, str
- Set: set, frozenset
- Mapping: dict
- Boolean: bool
- None: NoneType
5. What is a list, and how is it different from a tuple?
- List: Mutable, defined using square brackets [].
- Tuple: Immutable, defined using parentheses ().
6. What is PEP 8?
- PEP 8 is the style guide for writing Python code. It ensures readability and consistency.
7. What is the difference between append() and extend() in Python?
- append(): Adds an element to the end of the list as a single item.
- extend(): Adds elements of an iterable to the list.
8. What are *args and **kwargs?
- *args: Used to pass a variable number of positional arguments.
- **kwargs: Used to pass a variable number of keyword arguments.
9. What is a lambda function?
- A lambda function is an anonymous function expressed as lambda arguments: expression.
10. What is the difference between shallow copy and deep copy?
- Shallow copy: Creates a new object but inserts references to the original objects.
- Deep copy: Copies all objects recursively, creating independent copies.
11. How is exception handling done in Python?
- Exception handling is done using try-except blocks.
- Example:
- try: # risky code except ExceptionType: # handling code
12. What is the difference between is and ==?
- is: Checks identity (whether two objects are the same in memory).
- ==: Checks value equality (whether the values are the same).
13. What are Python decorators?
- Decorators are functions that modify the behavior of another function or method.
- They extend functionality without modifying the original code.
14. What is a generator in Python?
- A generator is a function that returns an iterator object using the yield keyword.
- It allows you to iterate one value at a time.
15. What is a module in Python?
- A module is a file containing Python code such as functions, classes, and variables.
- Modules can be imported and reused in other scripts.
16. How do you create a virtual environment in Python?
- To create a virtual environment: python -m venv myenv
- To activate: Windows: myenv\Scripts\activate, Mac/Linux: source myenv/bin/activate
17. What is GIL (Global Interpreter Lock)?
- GIL is a mutex in CPython that allows only one thread to execute Python bytecode at a time.
- It limits multi-threading performance in CPU-bound tasks.
18. What is a Python class and how do you create one?
- A Python class is a blueprint for creating objects.
- Example: class MyClass: def __init__(self, name): self.name = name
19. What is the difference between __str__() and __repr__()?
- __str__(): Provides a human-readable representation.
- __repr__(): Provides a formal string representation, often used for debugging.
20. What is list comprehension in Python?
- A concise way to create lists.
- Example: squares = [x**2 for x in range(10)]
21. What is the difference between a function and a method?
- Function: A standalone block of code.
- Method: A function associated with an object (e.g., a method in a class).
22. What is the difference between remove(), pop(), and del in lists?
- remove(): Removes the first matching element by value.
- pop(): Removes an element by index and returns it.
- del: Deletes an element by index or slices of elements.
23. How can you handle files in Python?
- Files are opened using the open() function.
- Example: with open('file.txt', 'r') as file: data = file.read()
- The with statement ensures the file is closed automatically.
24. What is monkey patching in Python?
- Monkey patching allows you to dynamically change a class or module at runtime.
- It is often used for testing purposes.
25. What are Python metaclasses?
- A metaclass defines the behavior of classes and is the class of a class.
- Metaclasses allow customization of class creation.
26. What is the pass statement?
- The pass statement is used as a placeholder for future code.
- It does nothing when executed but allows you to create empty code blocks.
27. How can you manage packages in Python?
- Python packages are managed using pip (Python Package Installer).
- Example: pip install package_name
28. What is the difference between Python 2 and Python 3?
- Python 3 has better Unicode support.
- Division of integers behaves differently (/ in Python 3 is float division, and // is integer division).
- Python 3 supports print() as a function, not a statement.
29. What is the use of super() in Python?
- super() is used to call the parent class method in a child class.
- It ensures proper method resolution order (MRO) in multiple inheritance.
30. How do you handle multiple exceptions in Python?
- Multiple exceptions can be handled using try-except blocks.
- Example: try: # code except (TypeError, ValueError): # handling code