Questions Courses
1. What is Java?
2. What are the main features of Java?
3. What is the difference between JDK, JRE, and JVM?
4. What is an object in Java?
5. What is a class in Java?
6. Explain inheritance in Java.
7. What is polymorphism in Java?
8. What are interfaces in Java?
9. What is the difference between an interface and an abstract class?
10. What are Java Collections?
11. What is the difference between ArrayList and LinkedList?
12. What is exception handling in Java?
13. What is a constructor in Java?
14. What are static members in Java?
15. What is the final keyword in Java?
16. What is multithreading in Java?
17. What is the Java Virtual Machine (JVM)?
18. What is the difference between == and equals() in Java?
19. What is garbage collection in Java?
20. What is the Singleton design pattern?
21. What is method overloading?
22. What is method overriding?
23. What is the use of the this keyword in Java?
24. What is the difference between an abstract class and an interface?
25. What are Java annotations?
26. What is a Java try-with-resources statement?
27. What are lambda expressions in Java?
28. What is the Stream API in Java?
29. What is the difference between ArrayList and Vector?
30. What is the volatile keyword in Java?
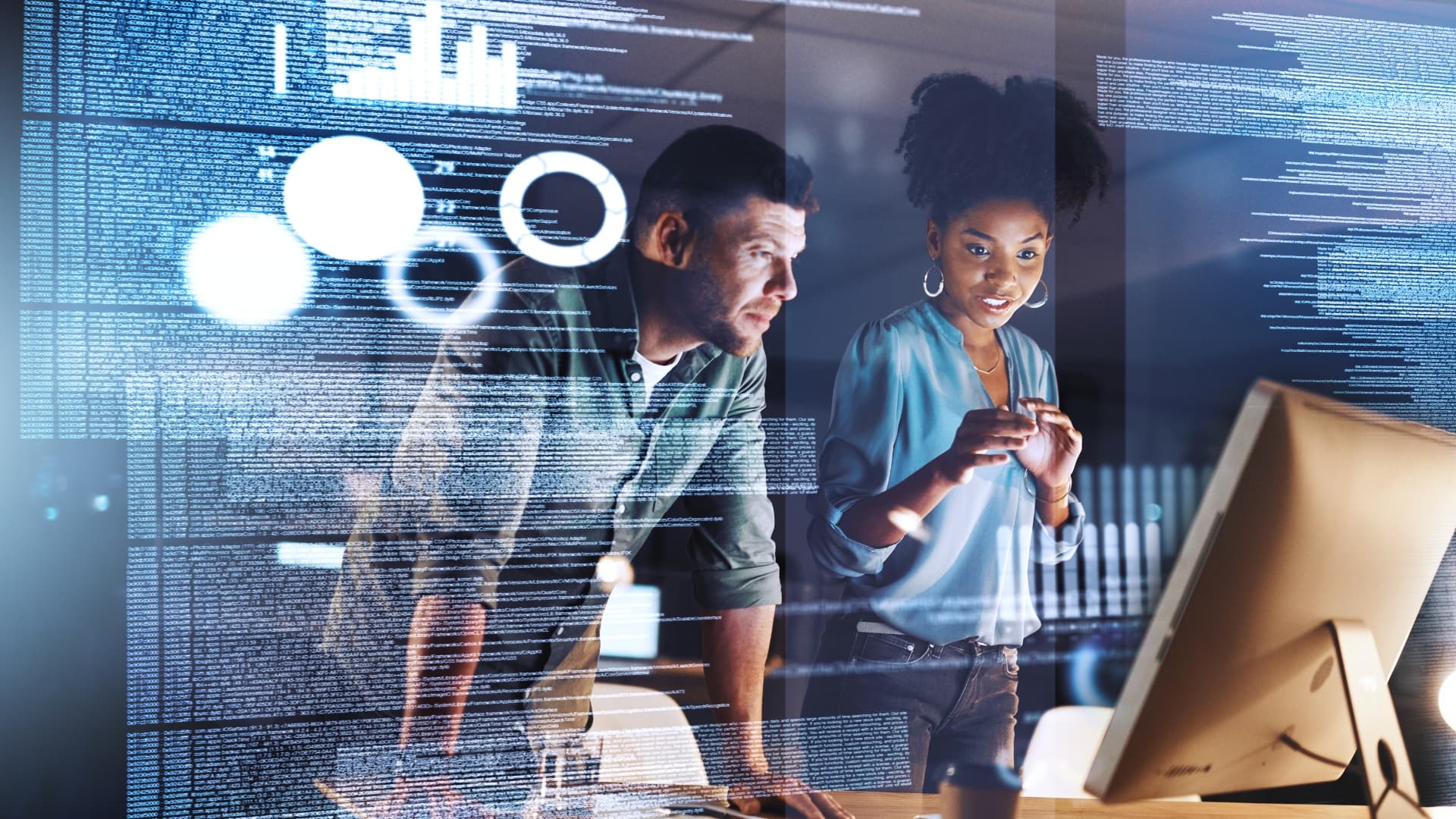
Java Interview Questions
Are you preparing for a Java interview? Here are 30 essential questions to help you succeed in your interview and demonstrate your mastery of Scrum.
Top Java Interview Questions & Answers
1. What is Java?
Java is a high-level, object-oriented programming language designed for platform independence and security.
2. What are the main features of Java?
Platform independence, object-oriented, robustness, security, multithreading, and rich API.
3. What is the difference between JDK, JRE, and JVM?
JDK (Java Development Kit) is for development, JRE (Java Runtime Environment) is for running applications, and JVM (Java Virtual Machine) executes Java bytecode.
4. What is an object in Java?
An object is an instance of a class, encapsulating data and behavior.
5. What is a class in Java?
A class is a blueprint for creating objects, defining attributes and methods.
6. Explain inheritance in Java.
Inheritance allows one class to inherit the properties and methods of another, promoting code reuse.
7. What is polymorphism in Java?
Polymorphism allows methods to do different things based on the object calling them, achieved through method overloading and overriding.
8. What are interfaces in Java?
An interface defines a contract of methods without implementation. Classes that implement it must provide concrete methods.
9. What is the difference between an interface and an abstract class?
Abstract classes can have both abstract and concrete methods and support single inheritance, while interfaces can only have abstract methods and support multiple inheritance.
10. What are Java Collections?
Java Collections is a framework for storing and manipulating groups of objects, including lists, sets, and maps.
11. What is the difference between ArrayList and LinkedList?
ArrayList provides fast random access and slower insertions, while LinkedList provides faster insertions and deletions but slower access.
12. What is exception handling in Java?
Exception handling is a mechanism to handle runtime errors using try, catch, finally, throw, and throws.
13. What is a constructor in Java?
A constructor initializes objects when they are created and has the same name as the class.
14. What are static members in Java?
Static members belong to the class rather than instances and can be accessed without creating an object.
15. What is the final keyword in Java?
The final keyword restricts modifications: final variables cannot be reassigned, final methods cannot be overridden, and final classes cannot be extended.
16. What is multithreading in Java?
Multithreading is the ability of Java to perform multiple tasks concurrently using threads.
17. What is the Java Virtual Machine (JVM)?
JVM is an abstract machine that executes Java bytecode, enabling platform independence.
18. What is the difference between == and equals() in Java?
== compares object references, while equals() compares the content of objects.
19. What is garbage collection in Java?
Garbage collection automatically reclaims memory by removing objects that are no longer referenced.
20. What is the Singleton design pattern?
The Singleton pattern ensures a class has only one instance and provides a global access point.
21. What is method overloading?
Method overloading allows multiple methods with the same name but different parameters in a class.
22. What is method overriding?
Method overriding provides a specific implementation of a method in a subclass that already exists in the superclass.
23. What is the use of the this keyword in Java?
this refers to the current instance of a class and is used to differentiate instance variables from parameters.
24. What is the difference between an abstract class and an interface?
An abstract class can have both abstract and concrete methods; an interface can only have abstract methods (prior to Java 8).
25. What are Java annotations?
Annotations provide metadata about the program and can be used for various purposes, including documentation and compile-time checking.
26. What is a Java try-with-resources statement?
This statement ensures that resources (like files) are closed automatically after use, improving resource management.
27. What are lambda expressions in Java?
Lambda expressions provide a concise way to represent functional interfaces (single abstract method interfaces) using an expression instead of an implementation.
28. What is the Stream API in Java?
The Stream API allows processing sequences of elements (like collections) in a functional style, enabling operations like filtering, mapping, and reducing.
29. What is the difference between ArrayList and Vector?
ArrayList is not synchronized and is generally faster; Vector is synchronized and thread-safe but slower due to overhead.
30. What is the volatile keyword in Java?
The volatile keyword indicates that a variable's value may be changed by different threads, ensuring visibility of changes to the variable across threads.