Questions Courses
1. What is .NET?
2. What are the different types of .NET frameworks?
3. What is the Common Language Runtime (CLR)?
4. What is the Global Assembly Cache (GAC)?
5. What are value types and reference types?
6. Explain the concept of delegates in C#.
7. What is an interface? How does it differ from an abstract class?
8. What is the purpose of the using statement in C#?
9. What is Entity Framework?
10. What is LINQ?
11. What are the differences between == and Equals() in C#?
12. What is garbage collection in .NET?
13. What is dependency injection?
14. What is middleware in ASP.NET Core?
15. What is the purpose of async and await in C#?
16. What is ASP.NET MVC?
17. What is the difference between REST and SOAP?
18. How do you handle exceptions in .NET?
19. What is caching, and how is it used in .NET?
20. Explain the concept of attributes in C#.
21. What is the difference between IEnumerable and IQueryable?
22. How do you implement logging in a .NET application?
23. What is a ViewModel in ASP.NET MVC?
24. What is the difference between a thread and a task in .NET?
25. How do you perform unit testing in .NET?
26. What is the role of the Startup class in ASP.NET Core?
27. What are the different types of authentication in ASP.NET?
28. What is the purpose of the Web.config file?
29. Explain the MVC design pattern.
30. What is the difference between asynchronous and parallel programming?
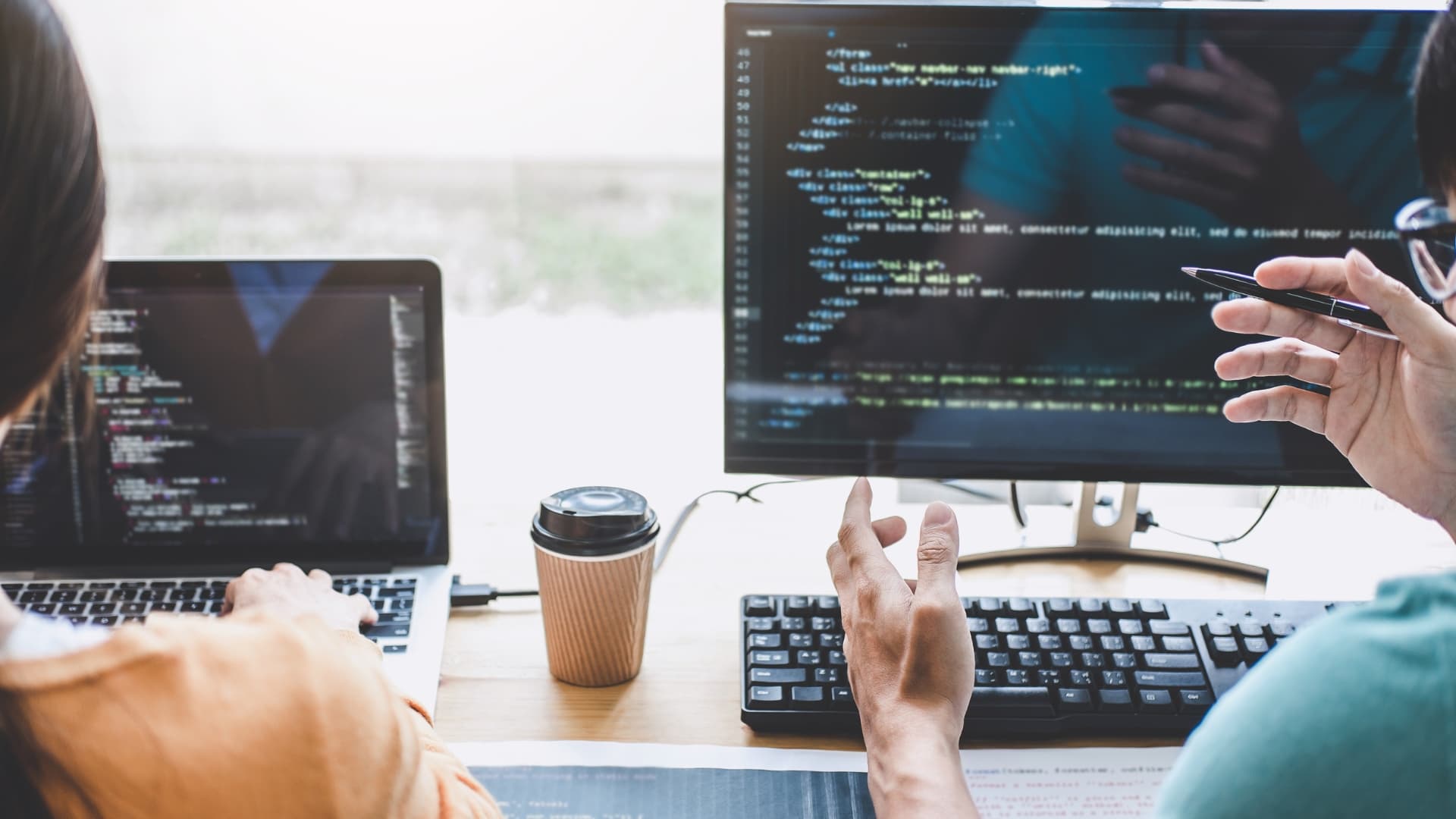
DotNet Interview Questions
Are you preparing for a DotNet interview? Here are 30 essential questions to help you succeed in your interview and demonstrate your mastery of Scrum.
Top DotNet Interview Questions & Answers
1. What is .NET?
- .NET is a software development framework by Microsoft that enables developers to create applications for Windows, web, and mobile platforms using various programming languages.
2. What are the different types of .NET frameworks?
- The primary types are:
- .NET Framework: Windows-only framework.
- .NET Core: Cross-platform framework.
- .NET 5/6: Unifies .NET Framework and .NET Core for building applications across platforms.
3. What is the Common Language Runtime (CLR)?
- CLR is the execution engine for .NET applications, managing memory, thread execution, and providing services like garbage collection and exception handling.
4. What is the Global Assembly Cache (GAC)?
- GAC is a machine-wide cache for storing .NET assemblies that are shared across multiple applications, allowing for versioning and side-by-side execution.
5. What are value types and reference types?
- Value Types: Store actual data (e.g., int, float, struct).
- Reference Types: Store references to data (e.g., class, array, string).
6. Explain the concept of delegates in C#.
- Delegates are type-safe function pointers that allow methods to be passed as parameters, enabling event handling and callback functionality.
7. What is an interface? How does it differ from an abstract class?
- An interface defines a contract without implementation, allowing multiple implementations.
- An abstract class can have both abstract methods (without implementation) and concrete methods (with implementation) and supports single inheritance.
8. What is the purpose of the using statement in C#?
- The using statement ensures that IDisposable objects are disposed of correctly and promptly, freeing up resources automatically.
9. What is Entity Framework?
- Entity Framework (EF) is an Object-Relational Mapping (ORM) framework for .NET that allows developers to work with databases using .NET objects instead of SQL queries.
10. What is LINQ?
- LINQ (Language Integrated Query) is a feature in .NET that provides a consistent way to query and manipulate data from various sources (e.g., collections, databases) using C# syntax.
11. What are the differences between == and Equals() in C#?
- == compares the references of two objects (for reference types) or values (for value types), while Equals() is a method that can be overridden to compare object content.
12. What is garbage collection in .NET?
- Garbage collection is the automatic memory management feature of the CLR, which reclaims memory occupied by objects that are no longer in use, preventing memory leaks.
13. What is dependency injection?
- Dependency injection is a design pattern used to achieve Inversion of Control (IoC) by injecting dependencies into a class rather than the class creating them, promoting decoupling and testability.
14. What is middleware in ASP.NET Core?
- Middleware is software that is assembled into an application pipeline to handle requests and responses, allowing for functionalities like logging, authentication, and error handling.
15. What is the purpose of async and await in C#?
- async and await are used to write asynchronous code, allowing operations to run in the background without blocking the main thread, improving application responsiveness.
16. What is ASP.NET MVC?
- ASP.NET MVC is a web application framework that follows the Model-View-Controller design pattern, allowing for separation of concerns, making applications easier to manage and test.
17. What is the difference between REST and SOAP?
- REST (Representational State Transfer) is an architectural style that uses HTTP requests for communication, typically with JSON or XML data.
- SOAP (Simple Object Access Protocol) is a protocol that relies on XML for message formatting and typically uses HTTP or SMTP for message negotiation and transmission.
18. How do you handle exceptions in .NET?
- Exceptions can be handled using try-catch blocks to catch and respond to exceptions, ensuring that the application can continue running or fail gracefully.
19. What is caching, and how is it used in .NET?
- Caching is storing data in memory to improve performance by reducing data retrieval times.
- In .NET, caching can be implemented using the MemoryCache class or distributed cache solutions like Redis.
20. Explain the concept of attributes in C#.
- Attributes are metadata added to program elements (classes, methods, properties) that provide additional information and can be accessed at runtime using reflection.
21. What is the difference between IEnumerable and IQueryable?
- IEnumerable is used for in-memory collections, allowing iteration, while IQueryable is designed for querying data from an external data source (like a database) and allows for building SQL queries.
22. How do you implement logging in a .NET application?
- Logging can be implemented using built-in frameworks like ILogger in ASP.NET Core or third-party libraries like Serilog or NLog, capturing different log levels (e.g., Information, Warning, Error).
23. What is a ViewModel in ASP.NET MVC?
- A ViewModel is a model specifically designed for a particular view in an ASP.NET MVC application, combining data from multiple models and including additional properties needed for the view.
24. What is the difference between a thread and a task in .NET?
- A thread is a lower-level construct that represents a single path of execution, while a task is a higher-level abstraction that represents an asynchronous operation, managed by the Task Parallel Library.
25. How do you perform unit testing in .NET?
- Unit testing in .NET can be done using testing frameworks like xUnit, NUnit, or MSTest, allowing developers to create and run tests to validate the functionality of code.
26. What is the role of the Startup class in ASP.NET Core?
- The Startup class is where the application configuration takes place, including service registration and middleware configuration in the ConfigureServices and Configure methods.
27. What are the different types of authentication in ASP.NET?
- Common authentication methods include:
- Basic Authentication: Simple username/password authentication.
- Forms Authentication: Custom login forms for web applications.
- Token-Based Authentication: Using tokens (e.g., JWT) for stateless authentication.
- OAuth/OpenID Connect: Delegated authorization using third-party identity providers.
28. What is the purpose of the Web.config file?
- Web.config is an XML configuration file used in ASP.NET applications to manage application settings, including database connections, authentication settings, and custom configurations.
29. Explain the MVC design pattern.
- The MVC pattern separates an application into three main components:
- Model: Represents the data and business logic.
- View: Represents the UI elements that display data to the user.
- Controller: Acts as an intermediary between the Model and View, handling user input and updating the Model.
30. What is the difference between asynchronous and parallel programming?
- Asynchronous programming is about non-blocking operations, allowing the program to continue executing while waiting for a task to complete.
- Parallel programming involves executing multiple tasks simultaneously, utilizing multiple threads to perform computations faster.